Combobox
Combobox is an accessible autocomplete input that enables merchants to filter a list of options and select one or more values.
Combobox component examples
Use when merchants can select one option from a predefined or editable list.
import {Listbox, Combobox, Icon} from '@shopify/polaris';
import {SearchIcon} from '@shopify/polaris-icons';
import {useState, useCallback, useMemo} from 'react';
function ComboboxExample() {
const deselectedOptions = useMemo(
() => [
{value: 'rustic', label: 'Rustic'},
{value: 'antique', label: 'Antique'},
{value: 'vinyl', label: 'Vinyl'},
{value: 'vintage', label: 'Vintage'},
{value: 'refurbished', label: 'Refurbished'},
],
[],
);
const [selectedOption, setSelectedOption] = useState<string | undefined>();
const [inputValue, setInputValue] = useState('');
const [options, setOptions] = useState(deselectedOptions);
const escapeSpecialRegExCharacters = useCallback(
(value: string) => value.replace(/[.*+?^${}()|[\]\\]/g, '\\$&'),
[],
);
const updateText = useCallback(
(value: string) => {
setInputValue(value);
if (value === '') {
setOptions(deselectedOptions);
return;
}
const filterRegex = new RegExp(escapeSpecialRegExCharacters(value), 'i');
const resultOptions = deselectedOptions.filter((option) =>
option.label.match(filterRegex),
);
setOptions(resultOptions);
},
[deselectedOptions, escapeSpecialRegExCharacters],
);
const updateSelection = useCallback(
(selected: string) => {
const matchedOption = options.find((option) => {
return option.value.match(selected);
});
setSelectedOption(selected);
setInputValue((matchedOption && matchedOption.label) || '');
},
[options],
);
const optionsMarkup =
options.length > 0
? options.map((option) => {
const {label, value} = option;
return (
<Listbox.Option
key={`${value}`}
value={value}
selected={selectedOption === value}
accessibilityLabel={label}
>
{label}
</Listbox.Option>
);
})
: null;
return (
<div style={{height: '225px'}}>
<Combobox
activator={
<Combobox.TextField
prefix={<Icon source={SearchIcon} />}
onChange={updateText}
label="Search tags"
labelHidden
value={inputValue}
placeholder="Search tags"
autoComplete="off"
/>
}
>
{options.length > 0 ? (
<Listbox onSelect={updateSelection}>{optionsMarkup}</Listbox>
) : null}
</Combobox>
</div>
);
}
Props
- activatorReact.ReactElement< & ( | | ) & ( | )>
The text field component to activate the Popover.
- allowMultiple?boolean
Allows more than one option to be selected.
- children?any
The content to display inside the popover.
- preferredPosition?'above' | 'below' | 'mostSpace' | 'cover'
The preferred direction to open the popover.
- willLoadMoreOptions?boolean
Whether or not more options are available to lazy load when the bottom of the listbox reached. Use the hasMoreResults boolean provided by the GraphQL API of the paginated data.
- height?string
Height to set on the Popover Pane.
- maxHeight?string
Callback fired when the bottom of the listbox is reached. Use to lazy load when listbox option data is paginated.
- minHeight?string
Min Height to set on the Popover Pane.
- onScrolledToBottom?() => void
Callback fired when the bottom of the lisbox is reached. Use to lazy load when listbox option data is paginated.
- onClose?() => void
Callback fired when the popover closes.
Anatomy
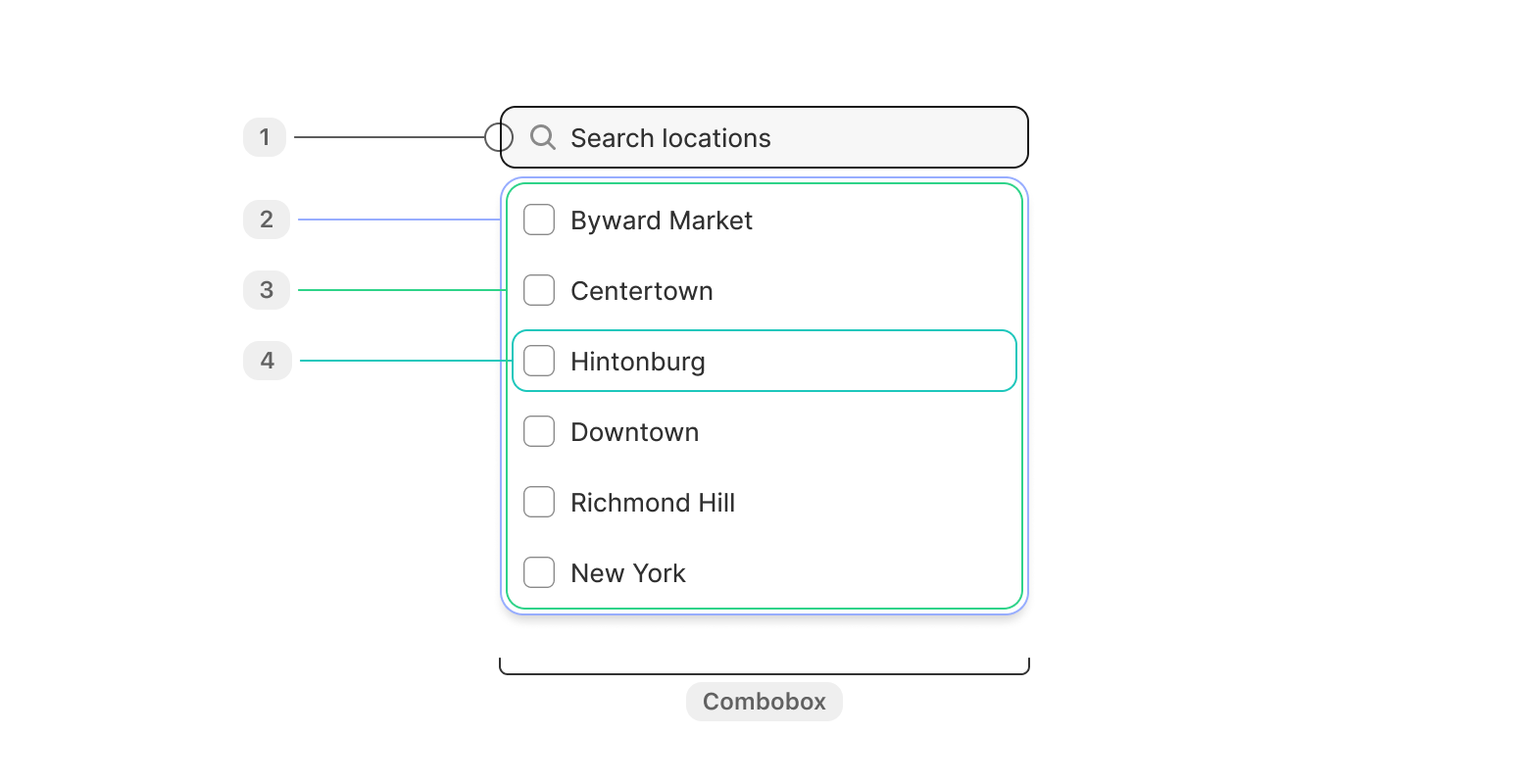
A combobox is made up of the following:
- TextField: A text input that activates a popover displaying a list of options. As merchants type in the text field, the list of options is filtered by the input value. Options replace or add to the input value when selected.
- Popover: An overlay containing a list of options.
- Listbox: A list of options to filter and select or deselect.
- Listbox.Option: The individual options to select or deselect. Check out the listbox component documentation to learn how to compose it with various content.
Best practices
The Combobox component should:
- Be clearly labeled so the merchant knows what kind of options will be available
- Not be used within a popover
- Indicate a loading state to the merchant while option data is being populated
- Order items in an intentional way so it’s easy for the merchant to find a specific value
Content guidelines
The input field for Combobox should follow the content guidelines for text fields.
Sorting and filtering
Sorting
Item order should be intentional. Order them so it’s easy for the merchant to find a specific value. Some ways you can do this:
- Sort options in alphabetical order
- Display options based on how frequently the merchant selects an option
If multiple options can be selected, move selected items to the top of the list. If this doesn’t work for your context, you can override this behavior.
Filtering
- By default, menu items are filtered based on whether or not they match the value of the textfield.
- Filters are not case-sensitive by default.
- You can apply custom filtering logic if the default behavior doesn’t make sense for your use case.
Patterns
Tags autocomplete
The tag multi-select input enables merchants to efficiently add or remove tags from a resource, like a product or an order. It uses the inline autocomplete combobox pattern to present merchants with an editable list of tags to browse and select from.
Related components
- For an input field without suggested options, use the text field component
- For a list of selectable options not linked to an input field, use the list box component
Accessibility
Structure
The Combobox component is based on the ARIA 1.2 combobox pattern. It is a combination of a single-line TextField and a Popover. The current implementation expects a Listbox component to be used.
The Combobox popover displays below the text field or other control by default so it is easy for merchants to discover and use. However, you can change the position with the preferredPosition prop.
Combobox features can be challenging for merchants with visual, motor, and cognitive disabilities. Even when they’re built using best practices, these features can be difficult to use with some assistive technologies. Merchants should always be able to search, enter data, or perform other activities without relying on the combobox.
- Use combobox as progressive enhancement to make the interface easier to use for most merchants.
- Require that merchants make a selection from the combobox to complete a task.
Keyboard support
- Give the combobox's text input keyboard focus with the tab key (or shift + tab when tabbing backwards)