This enables merchants to select a date or a date range from a list of preset dates.
How it helps merchants
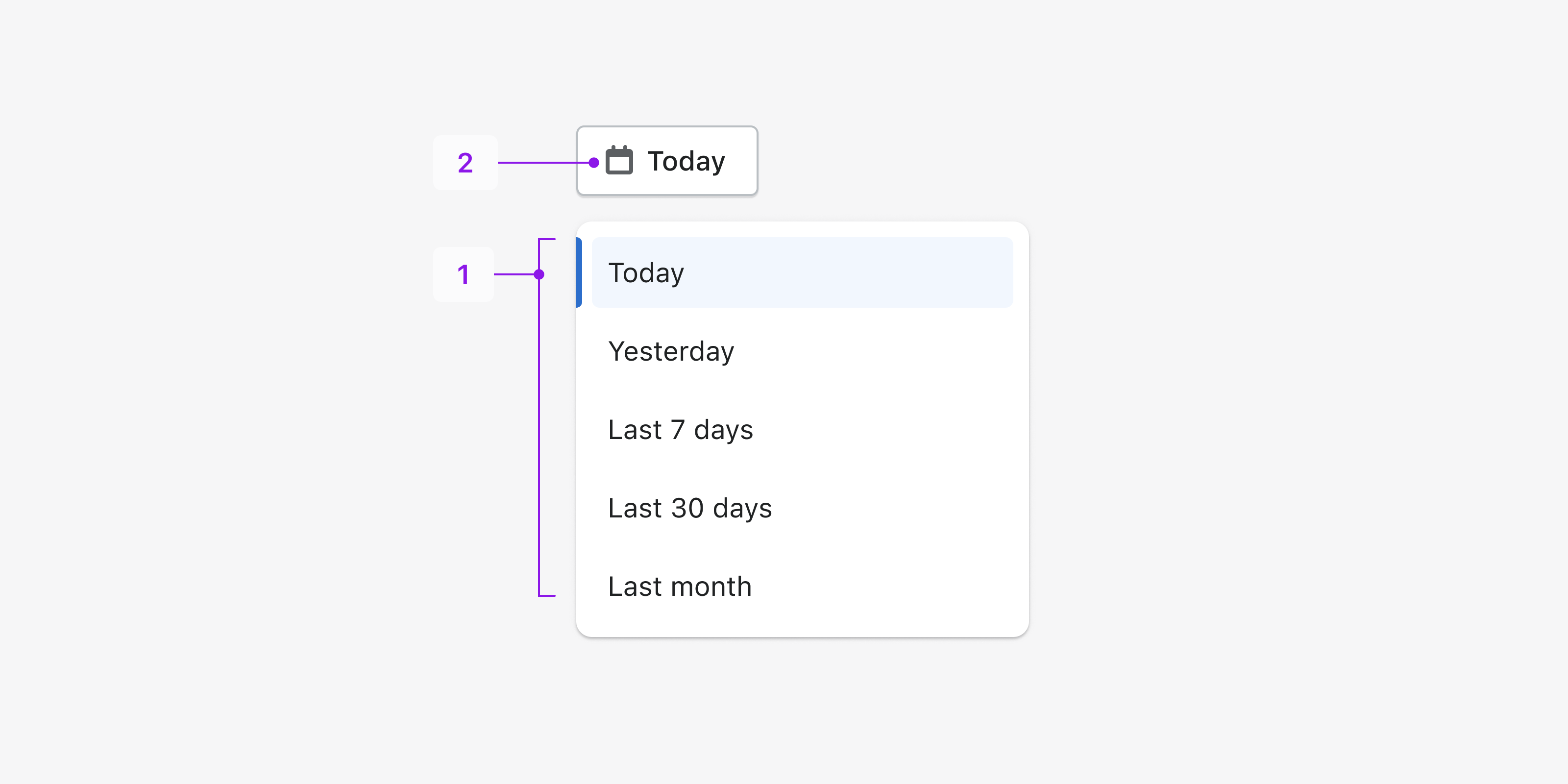
- The date list provides merchants with suggested dates. This makes date picking simpler when useful dates are predictable and custom dates aren’t necessary.
Use when merchants need to:
- Select from templated dates
- When a templated list of dates is sufficient for the merchant task, use the date list because it is a task that does not require in-depth filtering of historical information. Found in: Inbox app / Overview
Using this pattern
This pattern uses the Button, OptionList and Popover components.
// This example is for guidance purposes. Copying it will come with caveats.
function DateListPicker() {
const ranges = [
{
title: "No Date",
alias: "no-date",
period: null,
},
{
title: "Today",
alias: "today",
period: {
since: "today",
until: "today",
},
},
{
title: "Yesterday",
alias: "yesterday",
period: {
since: "yesterday",
until: "yesterday",
},
},
{
title: "Last 7 days",
alias: "last7days",
period: {
since: "-7d",
until: "-1d",
},
},
];
const [selected, setSelected] = useState(ranges[0]);
const [popoverActive, setPopoverActive] = useState(false);
return (
<Popover
autofocusTarget="none"
preferredAlignment="left"
preferInputActivator={false}
preferredPosition="below"
activator={
<Button
onClick={() => setPopoverActive(!popoverActive)}
icon={CalendarIcon}
>
{selected.title}
</Button>
}
active={popoverActive}
>
<OptionList
options={ranges.map((range) => ({
value: range.alias,
label: range.title,
}))}
selected={selected.alias}
onChange={(value) => {
setSelected(ranges.find((range) => range.alias === value[0]));
setPopoverActive(false);
}}
/>
</Popover>
)
}
Useful to know
-
In the button preview, set a default date range that a merchant will most likely use.
-
Single dates should be at the top of the list, followed by date ranges from smallest to largest ranges.
-
A date list can be modified to serve unique situations, like providing suggested search queries in the customer segment editor.
Related resources
- Programming timezones can be finicky. Get great tips in the article UTC is for everyone right?
- Learn about date formatting in the Grammar and mechanics guidelines.
- See how to craft effective button labels in the Actionable language guidelines.