How it helps merchants
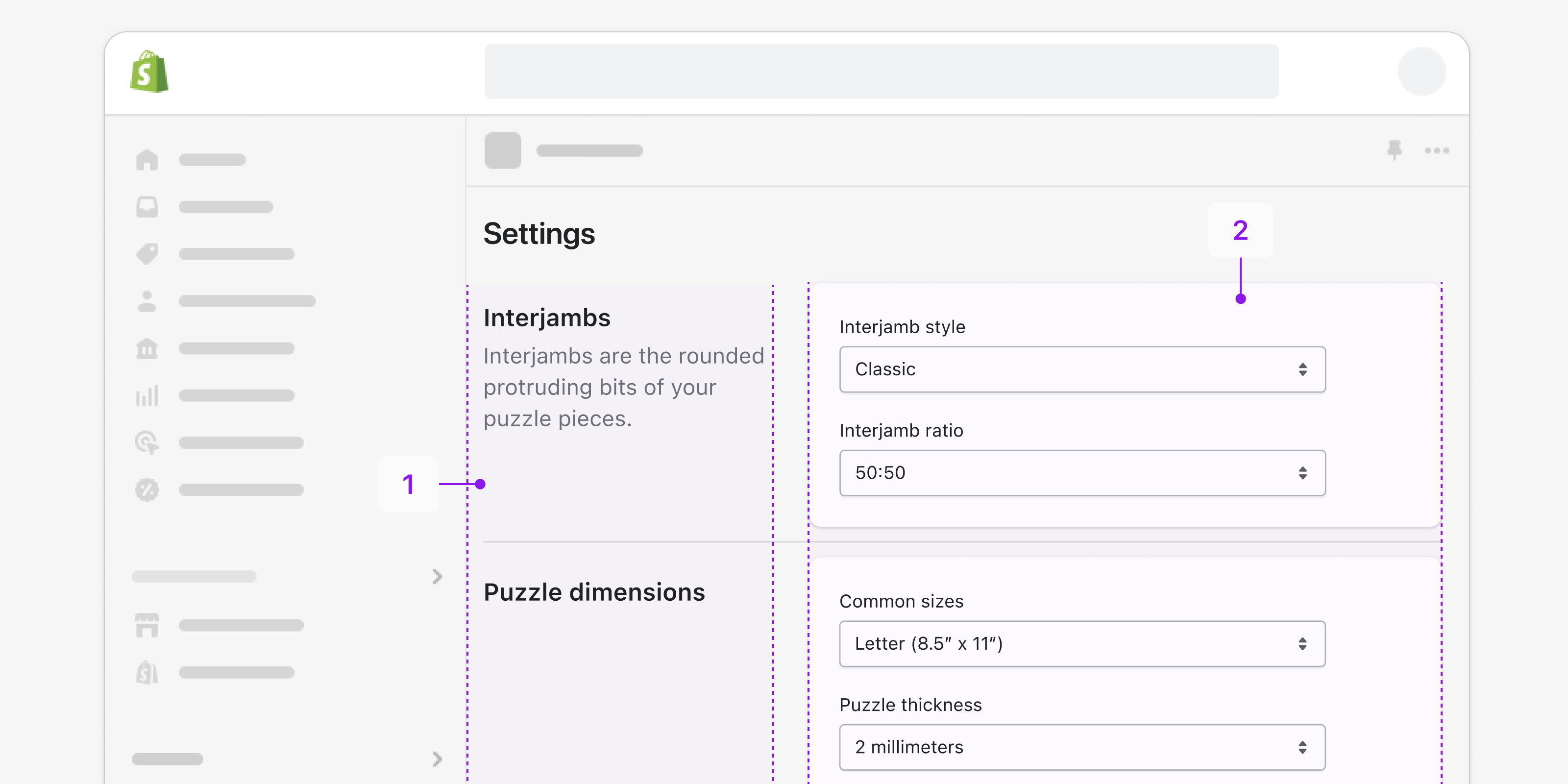
- In the left column, glanceable labels and descriptions are listed to make it easier for merchants to scan the page and quickly find what they are looking for.
- In the right column, settings are grouped in cards to make it easier for merchants to configure a setting after it's been found, or to configure multiple settings that might belong together.
Use when merchants need to:
- Find and change app settings
- This pattern is used specifically for finding and updating individual app settings within the Shopify admin.
Using this pattern
This pattern uses the BlockStack, Card, InlineGrid and Box components.
// This example is for guidance purposes. Copying it will come with caveats.
function AppSettingsLayoutExample() {
const { smUp } = useBreakpoints();
return (
<Page
divider
primaryAction={{ content: "View on your store", disabled: true }}
secondaryActions={[
{
content: "Duplicate",
accessibilityLabel: "Secondary action label",
onAction: () => alert("Duplicate action"),
},
]}
>
<BlockStack gap={{ xs: "800", sm: "400" }}>
<InlineGrid columns={{ xs: "1fr", md: "2fr 5fr" }} gap="400">
<Box
as="section"
paddingInlineStart={{ xs: 400, sm: 0 }}
paddingInlineEnd={{ xs: 400, sm: 0 }}
>
<BlockStack gap="400">
<Text as="h3" variant="headingMd">
InterJambs
</Text>
<Text as="p" variant="bodyMd">
Interjambs are the rounded protruding bits of your puzzlie piece
</Text>
</BlockStack>
</Box>
<Card roundedAbove="sm">
<BlockStack gap="400">
<TextField label="Interjamb style" />
<TextField label="Interjamb ratio" />
</BlockStack>
</Card>
</InlineGrid>
{smUp ? <Divider /> : null}
<InlineGrid columns={{ xs: "1fr", md: "2fr 5fr" }} gap="400">
<Box
as="section"
paddingInlineStart={{ xs: 400, sm: 0 }}
paddingInlineEnd={{ xs: 400, sm: 0 }}
>
<BlockStack gap="400">
<Text as="h3" variant="headingMd">
Dimensions
</Text>
<Text as="p" variant="bodyMd">
Interjambs are the rounded protruding bits of your puzzlie piece
</Text>
</BlockStack>
</Box>
<Card roundedAbove="sm">
<BlockStack gap="400">
<TextField label="Horizontal" />
<TextField label="Interjamb ratio" />
</BlockStack>
</Card>
</InlineGrid>
</BlockStack>
</Page>
)
}
Useful to know
-
Don't include a description unless it's helpful.
-
Place grouped settings within cards.
-
Stack all setting groups vertically on the page.
Related resources
- See another two-column layout in use in the Resource detail layout pattern.
- See a single-column layout in use in the Resource index layout pattern.
- Learn more about Layout in the app design guidelines.